C program to find hcf and lcm
C program to find hcf and lcm: The code below finds highest common factor and least common multiple of two integers. HCF is also known as greatest common divisor(GCD) or greatest common factor(gcf).
Download HCF and LCM program.
Output of program:
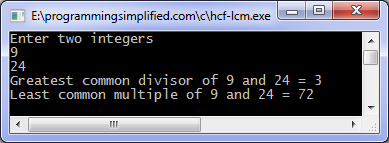
C programming code
#include <stdio.h>
int main() {
int a, b, x, y, t, gcd, lcm;
printf("Enter two integers\n");
scanf("%d%d", &x, &y);
a = x;
b = y;
while (b != 0) {
t = b;
b = a % b;
a = t;
}
gcd = a;
lcm = (x*y)/gcd;
printf("Greatest common divisor of %d and %d = %d\n", x, y, gcd);
printf("Least common multiple of %d and %d = %d\n", x, y, lcm);
return 0;
}
Output of program:
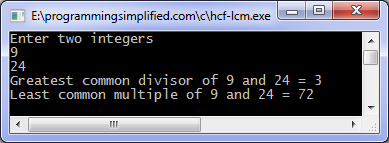
C program to find hcf and lcm using recursion
#include <stdio.h>
long gcd(long, long);
int main() {
long x, y, hcf, lcm;
printf("Enter two integers\n");
scanf("%ld%ld", &x, &y);
hcf = gcd(x, y);
lcm = (x*y)/hcf;
printf("Greatest common divisor of %ld and %ld = %ld\n", x, y, hcf);
printf("Least common multiple of %ld and %ld = %ld\n", x, y, lcm);
return 0;
}
long gcd(long a, long b) {
if (b == 0) {
return a;
}
else {
return gcd(b, a % b);
}
}
C program to find hcf and lcm using function
#include <stdio.h>
long gcd(long, long);
int main() {
long x, y, hcf, lcm;
printf("Enter two integers\n");
scanf("%ld%ld", &x, &y);
hcf = gcd(x, y);
lcm = (x*y)/hcf;
printf("Greatest common divisor of %ld and %ld = %ld\n", x, y, hcf);
printf("Least common multiple of %ld and %ld = %ld\n", x, y, lcm);
return 0;
}
long gcd(long x, long y) {
if (x == 0) {
return y;
}
while (y != 0) {
if (x > y) {
x = x - y;
}
else {
y = y - x;
}
}
return x;
}
No comments:
Post a Comment